44
|
How do I enable the cross link support ( rectangular )
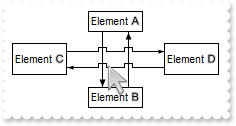
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element <sha ;;0>A",vtMissing,vtMissing);
var_Elements->Add("Element <sha ;;0>B",long(0),long(76));
EXSWIMLANELib::IElementPtr var_Element = var_Elements->Add("Element <sha ;;0>C",long(-76),long(32));
var_Element->PutAutoSize(VARIANT_FALSE);
var_Element->PutHeight(32);
EXSWIMLANELib::IElementPtr var_Element1 = var_Elements->Add("Element <sha ;;0>D",long(76),long(32));
var_Element1->PutAutoSize(VARIANT_FALSE);
var_Element1->PutHeight(32);
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
EXSWIMLANELib::ILinkPtr var_Link = var_Links->Add(spSwimLane1->GetElements()->GetItem(long(1)),spSwimLane1->GetElements()->GetItem(long(2)),vtMissing);
var_Link->PutStartPos(EXSWIMLANELib::CenterAlignment);
var_Link->PutEndPos(EXSWIMLANELib::CenterAlignment);
EXSWIMLANELib::ILinkPtr var_Link1 = var_Links->Add(spSwimLane1->GetElements()->GetItem(long(2)),spSwimLane1->GetElements()->GetItem(long(1)),vtMissing);
var_Link1->PutStartPos(EXSWIMLANELib::CenterAlignment);
var_Link1->PutEndPos(EXSWIMLANELib::CenterAlignment);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(3)),spSwimLane1->GetElements()->GetItem(long(4)),vtMissing);
EXSWIMLANELib::ILinkPtr var_Link2 = var_Links->Add(spSwimLane1->GetElements()->GetItem(long(4)),spSwimLane1->GetElements()->GetItem(long(3)),vtMissing);
var_Link2->PutStartPos(EXSWIMLANELib::LeftAlignment);
var_Link2->PutEndPos(EXSWIMLANELib::RightAlignment);
spSwimLane1->PutShowLinks(EXSWIMLANELib::ShowExtendedLinksEnum(EXSWIMLANELib::exShowCrossLinksRect | EXSWIMLANELib::exShowExtendedLinks));
|
43
|
How do I show a link frmo bottom to top, or reverse, not from left to right
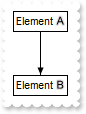
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element <sha ;;0>A",vtMissing,vtMissing);
var_Elements->Add("Element <sha ;;0>B",long(0),long(64));
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
EXSWIMLANELib::ILinkPtr var_Link = var_Links->Add(spSwimLane1->GetElements()->GetItem(long(1)),spSwimLane1->GetElements()->GetItem(long(2)),vtMissing);
var_Link->PutStartPos(EXSWIMLANELib::CenterAlignment);
var_Link->PutEndPos(EXSWIMLANELib::CenterAlignment);
|
42
|
Is it possible to control the links, so that they are always centered
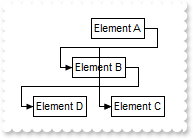
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element A",vtMissing,vtMissing)->PutID("A");
var_Elements->Add("Element B",vtMissing,vtMissing)->PutID("B");
var_Elements->Add("Element C",vtMissing,vtMissing)->PutID("C");
var_Elements->Add("Element D",vtMissing,vtMissing)->PutID("D");
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("B"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("D"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
spSwimLane1->PutShowLinks(EXSWIMLANELib::exShowLinks);
spSwimLane1->PutDefArrange(EXSWIMLANELib::exDefArrangeDir,long(1));
spSwimLane1->Arrange(vtMissing);
|
41
|
What options to align the elements do I have if I use Arrange method
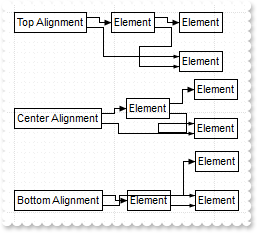
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->PutShowGridLines(VARIANT_TRUE);
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
_variant_t h1 = var_Elements->Add("Top Alignment",vtMissing,vtMissing)->GetID();
var_Elements->Add("Element",vtMissing,vtMissing);
var_Elements->Add("Element",vtMissing,vtMissing);
var_Elements->Add("Element",vtMissing,vtMissing);
_variant_t h2 = var_Elements->Add("Center Alignment",vtMissing,long(96))->GetID();
var_Elements->Add("Element",vtMissing,long(96));
var_Elements->Add("Element",vtMissing,long(96));
var_Elements->Add("Element",vtMissing,long(96));
_variant_t h3 = var_Elements->Add("Bottom Alignment",vtMissing,long(178))->GetID();
var_Elements->Add("Element",vtMissing,long(192));
var_Elements->Add("Element",vtMissing,long(192));
var_Elements->Add("Element",vtMissing,long(192));
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(1)),spSwimLane1->GetElements()->GetItem(long(2)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(1)),spSwimLane1->GetElements()->GetItem(long(3)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(2)),spSwimLane1->GetElements()->GetItem(long(4)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(2)),spSwimLane1->GetElements()->GetItem(long(3)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(5)),spSwimLane1->GetElements()->GetItem(long(6)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(5)),spSwimLane1->GetElements()->GetItem(long(7)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(6)),spSwimLane1->GetElements()->GetItem(long(8)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(6)),spSwimLane1->GetElements()->GetItem(long(7)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(9)),spSwimLane1->GetElements()->GetItem(long(10)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(9)),spSwimLane1->GetElements()->GetItem(long(11)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(10)),spSwimLane1->GetElements()->GetItem(long(12)),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(10)),spSwimLane1->GetElements()->GetItem(long(11)),vtMissing);
spSwimLane1->PutDefArrange(EXSWIMLANELib::exDefArrangeAlign,long(0));
spSwimLane1->Arrange(h1);
spSwimLane1->PutDefArrange(EXSWIMLANELib::exDefArrangeAlign,long(1));
spSwimLane1->Arrange(h2);
spSwimLane1->PutDefArrange(EXSWIMLANELib::exDefArrangeAlign,long(2));
spSwimLane1->Arrange(h3);
spSwimLane1->EndUpdate();
|
40
|
Is there an auto-arrange feature that will display the flow-chart centered and zoomed correctly after we are finished building it
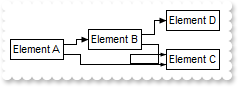
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element A",vtMissing,vtMissing)->PutID("A");
var_Elements->Add("Element B",vtMissing,vtMissing)->PutID("B");
var_Elements->Add("Element C",vtMissing,vtMissing)->PutID("C");
var_Elements->Add("Element D",vtMissing,vtMissing)->PutID("D");
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("B"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("D"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
spSwimLane1->Arrange(vtMissing);
|
39
|
Is it possible to change the distance between elements, when calling the Arrange method
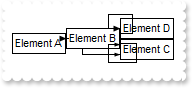
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element A",vtMissing,vtMissing)->PutID("A");
var_Elements->Add("Element B",vtMissing,vtMissing)->PutID("B");
var_Elements->Add("Element C",vtMissing,vtMissing)->PutID("C");
var_Elements->Add("Element D",vtMissing,vtMissing)->PutID("D");
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("B"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("D"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
spSwimLane1->PutDefArrange(EXSWIMLANELib::exDefArrangeDX,long(0));
spSwimLane1->PutDefArrange(EXSWIMLANELib::exDefArrangeDY,long(0));
spSwimLane1->Arrange(vtMissing);
|
38
|
How do I organize vertically the elements
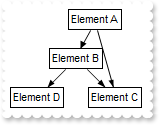
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element A",vtMissing,vtMissing)->PutID("A");
var_Elements->Add("Element B",vtMissing,vtMissing)->PutID("B");
var_Elements->Add("Element C",vtMissing,vtMissing)->PutID("C");
var_Elements->Add("Element D",vtMissing,vtMissing)->PutID("D");
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("B"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("A"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("D"),vtMissing);
var_Links->Add(spSwimLane1->GetElements()->GetItem("B"),spSwimLane1->GetElements()->GetItem("C"),vtMissing);
spSwimLane1->PutShowLinksType(EXSWIMLANELib::exLinkStraight);
spSwimLane1->PutDefArrange(EXSWIMLANELib::exDefArrangeDir,long(1));
spSwimLane1->Arrange(vtMissing);
|
37
|
How can I add programatically a link
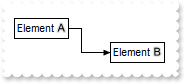
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element <sha ;;0>A",vtMissing,vtMissing);
var_Elements->Add("Element <sha ;;0>B",long(96),long(24));
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(1)),spSwimLane1->GetElements()->GetItem(long(2)),vtMissing);
|
36
|
How do I get the link from the cursor
// MouseMove event - Occurs when the user moves the mouse.
void OnMouseMoveSwimLane1(short Button,short Shift,long X,long Y)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
ObjectPtr l = ((ObjectPtr)(spSwimLane1->GetLinkFromPoint(-1,-1)));
OutputDebugStringW( L"l" );
}
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element <sha ;;0>A",vtMissing,vtMissing);
var_Elements->Add("Element <sha ;;0>B",long(96),long(24));
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(1)),spSwimLane1->GetElements()->GetItem(long(2)),vtMissing);
|
35
|
Is there a way to create a link which has the same start and end element
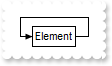
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element",vtMissing,vtMissing);
EXSWIMLANELib::ILinksPtr var_Links = spSwimLane1->GetLinks();
var_Links->Add(spSwimLane1->GetElements()->GetItem(long(1)),spSwimLane1->GetElements()->GetItem(long(1)),vtMissing);
|
34
|
How can I show the pool's caption not-rotated or mirrored
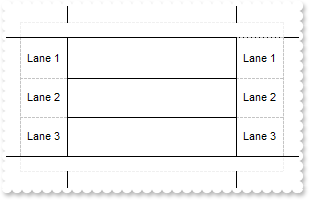
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->PutAllowDesignHeader(EXSWIMLANELib::exDesignHeaderSingle);
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-150),long(-150),long(300),long(150));
var_Pool->PutHeaderCaptionSupportRotate(VARIANT_FALSE);
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderLeft,48);
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderRight,48);
var_Pool->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(3);
spSwimLane1->EndUpdate();
|
33
|
How can I prevent creating sub-lanes or sub-phases
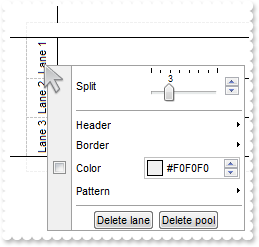
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->PutAllowDesignHeader(EXSWIMLANELib::exDesignHeaderSingle);
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-150),long(-150),long(300),long(150));
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderLeft,32);
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderRight,32);
var_Pool->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(3);
spSwimLane1->EndUpdate();
|
32
|
How do I show a complete frame/border around the header
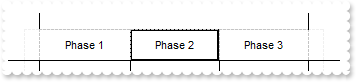
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-150),long(-150),long(300),long(150));
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderTop,32);
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderBottom,32);
EXSWIMLANELib::ILanesPtr var_Lanes = var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren();
var_Lanes->PutCount(3);
var_Lanes->GetItem(long(1))->GetHeader()->GetPattern()->PutType(EXSWIMLANELib::exPatternFrameThick);
spSwimLane1->EndUpdate();
|
31
|
How do I show a complete frame/border around the lane
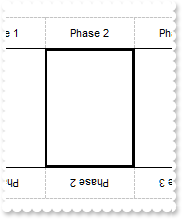
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-150),long(-150),long(300),long(150));
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderTop,32);
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderBottom,32);
EXSWIMLANELib::ILanesPtr var_Lanes = var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren();
var_Lanes->PutCount(3);
var_Lanes->GetItem(long(1))->GetPattern()->PutType(EXSWIMLANELib::exPatternFrameThick);
spSwimLane1->EndUpdate();
|
30
|
How can I define sub-lanes
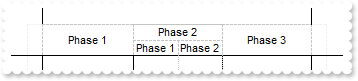
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-150),long(-150),long(300),long(250));
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderTop,32);
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderBottom,32);
EXSWIMLANELib::ILanesPtr var_Lanes = var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren();
var_Lanes->PutCount(3);
var_Lanes->GetItem(long(1))->GetChildren()->PutCount(2);
spSwimLane1->EndUpdate();
|
29
|
How can I add default lanes when the user creates a new pool
// AddPool event - A new pool has been added to the surface.
void OnAddPoolSwimLane1(LPDISPATCH Pool)
{
// Pool.HeaderSize(1) = 24
// Pool.HeaderVisible(0) = False
// Pool.HeaderVisible(1) = True
// Pool.HeaderVisible(2) = False
// Pool.HeaderVisible(3) = False
// Pool.Lane(1).Children.Count = 2
}
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->GetPools()->Add(long(-100),long(-100),long(250),long(250));
spSwimLane1->EndUpdate();
|
28
|
How do I prevent showing headers when the user creates new pools
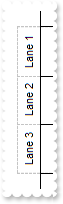
// AddPool event - A new pool has been added to the surface.
void OnAddPoolSwimLane1(LPDISPATCH Pool)
{
}
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->GetPools()->Add(long(-100),long(-100),long(250),long(250))->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(3);
spSwimLane1->EndUpdate();
|
27
|
How can I display icons on headers
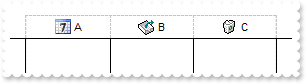
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-100),long(-100),long(250),long(250));
var_Pool->PutHeaderVisible(EXSWIMLANELib::exHeaderLeft,VARIANT_FALSE);
var_Pool->PutHeaderVisible(EXSWIMLANELib::exHeaderRight,VARIANT_FALSE);
var_Pool->PutHeaderVisible(EXSWIMLANELib::exHeaderBottom,VARIANT_FALSE);
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderTop,24);
EXSWIMLANELib::ILanesPtr var_Lanes = var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren();
var_Lanes->PutCount(3);
var_Lanes->GetItem(long(0))->GetHeader()->PutCaption(L"<img>1</img> A");
var_Lanes->GetItem(long(1))->GetHeader()->PutCaption(L"<img>2</img> B");
var_Lanes->GetItem(long(2))->GetHeader()->PutCaption(L"<img>3</img> C");
spSwimLane1->EndUpdate();
|
26
|
How do I change the Lane and Phase strings being displayed on the lanes
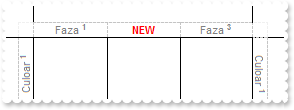
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->PutDefPoolHeaderCaptionFormat(VARIANT_TRUE,L"<fgcolor 808080>Faza <off -4><b><font ;6>%i");
spSwimLane1->PutDefPoolHeaderCaptionFormat(VARIANT_FALSE,L"<fgcolor 808080>Culoar <off -4><b><font ;6>%i");
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-100),long(-100),long(250),long(250));
var_Pool->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(3);
var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren()->PutCount(3);
var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren()->GetItem(long(1))->GetHeader()->PutCaption(L"<fgcolor FF0000><b>NEW");
spSwimLane1->EndUpdate();
|
25
|
How can I enlarge the node while AutoSize property is True (method 2)
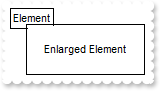
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutBackground(EXSWIMLANELib::exElementBackColor,RGB(255,255,255));
EXSWIMLANELib::IElementPtr var_Element = spSwimLane1->GetElements()->Add("Element",long(0),long(0))->Copy(vtMissing,vtMissing);
var_Element->PutCaption(L"Enlarged Element");
var_Element->PutInflateSize(16);
|
24
|
How can I enlarge the node while AutoSize property is True (method 1)
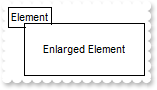
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutBackground(EXSWIMLANELib::exElementBackColor,RGB(255,255,255));
EXSWIMLANELib::IElementPtr var_Element = spSwimLane1->GetElements()->Add("Element",long(0),long(0))->Copy(vtMissing,vtMissing);
var_Element->PutCaption(L"Enlarged Element");
var_Element->PutPadding(EXSWIMLANELib::exPaddingAll,16);
|
23
|
How can I get the lane of the element
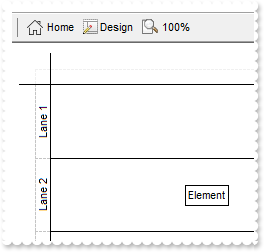
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->GetPools()->Add(long(-150),long(-150),long(500),long(250))->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(3);
EXSWIMLANELib::IElementPtr var_Element = spSwimLane1->GetElements()->Add("Element",vtMissing,vtMissing);
var_Element->CenterOnLane(VARIANT_FALSE);
_variant_t lane = var_Element->GetLaneID(VARIANT_FALSE);
OutputDebugStringW( spSwimLane1->GetLaneByID(lane)->GetHeader()->GetCaption() );
spSwimLane1->EndUpdate();
|
22
|
How can I define a different shape for elements
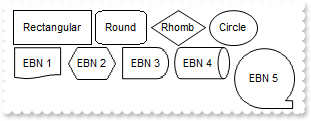
// AddElement event - A new element has been added to the surface.
void OnAddElementSwimLane1(LPDISPATCH Element)
{
}
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IAppearancePtr var_Appearance = spSwimLane1->GetVisualAppearance();
var_Appearance->Add(1,_bstr_t("gBFLBCJwBAEHhEJAAChABAQDg6AADACAxRDQNABQKAAzQFAYahuHKGAAGEaBYgmFgAQhFcZQSKUOQTDKMIziYBYJhEMQwDiAUIjIK8IhkGIcZAGIBJCjWGodQLOEgwH") +
"IERQjEyUJAGGYqEjaO41SpAdI0PCxUScACnDQiegJRgmQ4cTJSchSAKENx1JaeYReewwAqNd5TRwNIiydZUIhqGC1YRte4ZWiCWoJVzSVDLfbgAT4X60IIlCi5Mi6MIg" +
"DZJFDUNLUdRBMKNJ7qagaWoWOaQRbmAQQTRYLQ/TiEXZDQRAAyLJIWVRQWTAAKVbw1LiEcznGCcejzIIDaZpOh4DplWzTOgALjta4IRpvNwAbIAF4gBqsLyXXLcdQyOR" +
"MiwbxZgSDhgnQbJFC6F5JhECA8CADwIgMeQnmoQJlh0eAfGcXYZjwccOHiWx/moAJ6jed4DC2dJnnmQRxBcKABBoWAcHULZLHyV4mmGOx0FmdpZAMAgQhEQBpBiRxBlQ" +
"cgZFUdAMEYAxQDECBNgaUYglkCQKBGZANk0QgBgQXAQgcGI0FwJAHA6WI+DGDAjCiVg0g2Yw4mYNg7E0eJ1H+DpkAiYhBg6JApBYRMvDkJhGhKJIImEA4QledZ8H+FJl" +
"EEQhWBAJa8loRoCgkdhYBAExZHQKIXmUYRCFQI5mgWfZ4hMJpJC4boTGcCY0m0QJVBAIh2EIZZ5H4VIVAqeZ1H8M5oAmSg7g8TR1n4fQrmUQZCgqF5eEmYhYBgKQJiCD" +
"4PmaegKhWJZnAQDZkhqaYKFocYnGadZ8h+KJoiGQhfEIURAmKEAYAgeo1H8OZrAoeoyhGKYngaHInmuCpOjmKAkHMHo+iiSZxmKQoXkGCwWigRQlnqLh7jKSh7DYUYzm" +
"0CgAk+DIrFCAo3i6LYKnKWYuk6Wp4keKIqDsLh7BYLhJmaZo5mcYAijAOZtFsXpajubZLG6co8CSShtnYeJrGeVpzjwbxLnafI/mcK5ZnmPgtGuap+j6cIMB8CocDAK4" +
"3AaQAwjwJwQkSaZsDcBI7jETBHBqRhxkibwWkCMQsgcG5Ii2TB+nkN5yEyJwjkqcJMh8DpKDKTIrB2TBmnyGwFkwMw8mcMpOmQbJbBiTwziyUxBDsc5sD8QJRnQKprEW" +
"Tg0g0DxNlKMpsF8RpSHUTQ7BuUp0n0VxZi4MxrDSJ40DYDYHGKWJ2E2FxmyADYnGqMRBgeYh0FOLh9kccpcncJsVw7RdjvA2M4eovRmCSFkMwQIoAQEBA");
var_Appearance->Add(2,_bstr_t("gBFLBCJwBAEHhEJAAChABAIDg6AADACAxRDQNABQKAAzQFAYaBmG6GAAGEaBYgmFgAQhFcZQSKUOQTDKMIziYBYJhEMQ1DAAUIjKLMIhmGAYJAGIBJCjWGodQLOEgwH") +
"IERQjEyUpIyVAkYw5HqNaQjICoJoSOwAU47IaCIAFJSpCygKKgSjhRpoAAyU5IUgVPC4XSbD6zYDqapaTheEQyDSJNr3DCMUTlFYYLrmaQKMhqZJhWjbFSWXUpJ4pSrL" +
"MIyfTwZRJGzIK7jabLIjaP6GUDhVaYbRUL46CiEb5AAJsVpCCZrYRJOYVdQeDYbQCNLDhHQIDqaA6kZRAdQ2bpMBznPSvbovDgLWhGYqhbLCNibRC+d4HNrCYIlCjpMw" +
"3CYGIOHOMRsm0OxFjSZppneHQNFcSwRAOaprjqY4dgYe4VgGWwADucRuHYCpdByYROH8AAOC8D4NgEXwYBWEZyGAWoHg2MRCECBAkFAGhGDGUB1ByBhQkUIAPgCRAMEI" +
"ExhIuNhCEKBAlnAcwQgAYQXAIIRWEUFwJAGQ42CiCoihiLgugsYwDjSZ4MkICJWCaDZjgiZg6EIQQInSV4PmKeIyEKEBkBQDhEgwZBzjSd4SlEQJgk+E5khkRhIhSZA5" +
"FIUoVGWCQkl+FpkliTJnhXSYaFaGJOlEIhmC2ZoJgIZIaiWGYuG62IpDyT4TGSM5eGyCpmCnPIcCeQg+H6HZmnoAh6iAaBDjSL4hkUCgmgKHhlHmeIPh8BwKAqEohGkO" +
"gOHCI4pAmMoMhUKByj6CQUCkWoGhSKJpGkJoQiOJR6G4cYTmcSQuiKKRqEqPovhmBIJiCN4YEACQGFsN5lliToyiwbAKkKQoSGcWQ2HKERrhiJJvjLUR8leL5sDsBpQi" +
"UZZpDaO4eAACZWh+LYtDsSpejQbmJnIEAuEsLJvECRgLiSH46m2W4Gl+OxpFqWo0ECSR7lQaI9m8C4+nqO4uAIHp+B4MAJmcAAeC+YgenmPwwHwHInkKRYMDaWIQGmeh" +
"3AmPpxDsCJHkWbhBAIdZDmMGZXBmRZMHwVwikUIwam0MAMC4awiEmOYyHsXwkkIMp5jMLBMDKaRiiqEBsmkNwpkwc5MyiHRrEKfxCiobIaFsQgvmsTBvD2SJuAwNBYkK" +
"Sh6GsRYbDUDQ7EyVZmEwDwFiKdQ6BQf5XnYDRjD6IIujIfppBgKRcl6BZZnYXYLBWLYpn0KpglqLAtAIZoOneBsZw9RJjnQ+AYQAgCAg=");
var_Appearance->Add(3,_bstr_t("gBFLBCJwBAEHhEJAAChABQsMACAADACAxRDQNABQKAAzQFAYawLBgABhGUZoJhUAIIRZGMIjFDcEwxC6NIpAWCYQgUNQCQiNAzxAKQchhD4ZAIkGY4ZhyA42SBAcbyD") +
"CEShoGqPRhmCg5UjmM4oQLSFDULC0fSBBIYaSGEY5QoqIYfArGQYQTRUPyUdoOBIACwLChWLA1CTZdowSKoYTXBq3IgqerIapmSYaV7YNh3VY1IR/JSVJYlaYJDoyNI4" +
"ThHc5xVLtfSfAiiJrxOKcTAAFJ0QKFUbRUz+OgBTpvaZxagaSpSG5WRpWEI5PAdN4zTa7YDsS67FiSG5oWpEFq2eAGdzlVLMMqhG5gAxqNKzbLfMCXfJIbyrBIcYLoXI" +
"tCqFYskMTwxlgAY+haFZRlQZQ4HwHR3AoMx5jEch2lMfZGl8eY+jYXBvBsAAHFeRQDmuRR8nsSI2CoEgIEIRQBiQYwdAcUZIGUUIQhGGAGECRAhDYChGFERAMCgQheEc" +
"GQgHkEAwkICx2BCFoAj+B5iAiBgigiYhIgid4JkIQBwm2ChijiKgsguYpokYLYMmKQIiDYDgjgEAg6g6Y5InYPoPmOIAiEKEJFgkFIvhGYwIlIMoSTmAhOGgJJJC4UoS" +
"iUSIcncKZlp8WoOAOAxeF6FBlEkNhPGcZgJhYU4ZiMMweGoDAmkGfhuhWJoJDgIIUCYeZWGGHYkhkWhuB+ZYhEIcIUmcKZSHeIJmgoFgyCeaIBgKCoYCgGYSHiI5oEoQ" +
"gsiKBgKCCH4SOAfoGiQaZKPmJ5jGCIJniiZgpk6DonGkKomgOHpnjqFoCiaawKioSYXg0eoGi6KZrBqIo6hgEIal6GosiwCpuDIBgsEqDJniYa4bVuFYkDqepKjCLQJi" +
"iR4XCyawGk+JpGgsZIXjabRbEKV4disSYwDCH5OlsIpWjmaA5HKUomCYOp+l6OgkhoUoWiMLpbH6bw7G0KRynOHYuGuXobhWB4MAaaoaimO52naB4hnkYInhERYLBAGA" +
"hnECw+H2BwhkmSg7kMMIqHyb49hAECAg=");
var_Appearance->Add(4,_bstr_t("gBFLBCJwBAEHhEJAAChABOIDg6AADACAxRDQNABQKAAzQFAYaBqGCGAAGEZRSgmFgAQhFcZQTCsBw7DCEYxjOAwFgmEQxDIOIBQSKYcwiGQaRrkIYgEiONoaR7HchSF") +
"IcIxPFKRBhkKYocjyG5GRoBcIyXAcRSYAB2Q4ESoKShePw5UDTcCzHR8RxEDKNRLpOQbDgOUZTWbVUaDKIsXzZCKHbZhG4YRp6CZKRpYEB1bYtKTRKqLKrpeTbOoSHol" +
"TbiIATTYlG4THyEZQrDIaDjOSIXZaGFzwSDWGgBJ60bRwHIaAxGWoHRxfEZVfBONQHMSBc5xa64JyHAImWrcUbWfaYYZ0AC6MRkK8cXABPQANixKZsCizE4DDbTYzjRh" +
"eC2F4nnEOJRG0BRXEWOICEocByiyCJTiQOJVGYIQ8gofpDgsG5uF+ah6D2Xp+GGP5gkCfwRHofwOnuSB2heVpZnIUZQhAIQJBQBoRgwdAdEcIYVEIQhGGAMQDBCBJYEU" +
"GgNhCEMcHsXIkCIAAMIILQWAkAZDjYJIJiIWIeCqCRMHiNI/guYwIkYMoMmMSJWDaDZjgiTIzg6Yo4iIPoPGIdAmECChiFKXhGAQJIAmISgQCSSQ2E6E5lAkRhShSZRJ" +
"FQOoVkCaRkAqFpQhIPhehCY54hYQoRCOYo+GaExOnmJhghqZhJjIYYbmaaJ0l+HJkiCYgtDGY5RkIegfmeSY6GOD4MnoBh1D2aBJkYbYhmcCgigaIhOloEJ3h0aATD4b" +
"gWCkQoehaGAnniHhNgwKI6HaConmiSYCF4I5niGQoLDmaoAiKKoaE6eJ2H+HZnFoeohigKwqCwGItmqA4ejIHgrkIHo+iuEA4h6PGMmIHo1i0LIKk6SoMCSWwcm2LAki" +
"sAAAgmQgLFYcw3myaxmkmG4GgsdIXjebYjjaVQIC0eQFAONADguBpqjaaIKlwGIuG4GwOkWOYAAqDoljqbIrmIJ41m4O5iGYK5FAwBp0ikag7mqbI+GoCwyEyPgpBCQw" +
"Oj0KRBGKFgpnAbAjXWMQ8EaeIuG6a5SHWHZxFyBoIjEbA8FQCJJmiZAmmOORwHyCwXgkbwqn8LYoDKOZNAmJpinyOzTm8aZrBWH5QhGAxCCedBMm8L5Fi+fAEFCPgvks" +
"Vp0h8NQBn8RpLi7NQKBALxLHyF43EaDRPDOMoxkqXp4kYaRLlMTQIDWbQeguR5iluBxCBgNBCH6dIJnaSZjF+LQZgGQSh5DOFEa4dQsi5GILsaA8ReBuHoEYZ4ZwkDFF" +
"wJEYo2grjcHOFUAoFA7gJFqFEDYrQyilC+PwNgyRBBLH4C8FIzxiD8CeBYEgUQ9CtAiEwYgtQyDqDWPUTjBhXBrCmAcKoJQ6iGF6DEMA8BpieA4BgMQdxDg9GSOkLQgR" +
"CjhHiDMV4Ewfh3AoGULIiBujRAuJ0c4+gEi4ASOkRAfhWgjCKKEFACCAg");
var_Appearance->Add(5,_bstr_t("gBFLBCJwBAEHhEJAAChABGgDg6AADACAxRDQNABQKAAzQFAYZBwGiGAAGEaRWgmFgAQhFcZQSKUOQTDKMIziYBYJAKCQ3DTJUBjIKcIhiGsgAzAJIUaw1DqGY7nKZoR") +
"ioAIoSAMM7DCKUQSLGyQZqBSCQGjsAI+OyHAiABSNJwtHygIRoKqqHg2PoiSAEUZhdRlHShKSqLQiaIRSDUJZsW5EIyjBZ8EznOqbJApOKrCgOTYaWbUdSxPTEUwTFiX" +
"JgmKRKIoiOAAY7IEgSFLsThrIKha5yDI4bxyAwXQjeYABbY0E4HQarcqzCrKGp/HYJXyAGgQHYkB5JAaQMBtGpoJrCeKQXDdVyXFwdET1BLZQA2HeUTTRVCScqnaTMZh" +
"8DgGxniKfYbngAxFkmVJAnSdougwDhRlMGZ1GEPR9HQapDGWWhyCMe4ugQDx9naYRvm6cQfAUJ47m8cofBYAZOGCHg2mETgCBCERAGkGJHEGVByBkUwUCSIgChAMQIE2" +
"BJRiQVQDAoEIWlWIxOgGBBcGyBQYlQXAkAcDpYh4KYKCKKI2CyC5ijONJvgySZ4lQWINkKCJmCWDpjkQaJPg+UA4joMYQiQI4eEYHAkgAYhKBgJJCh43JlAkEgwhSJA5" +
"E4VoVE6UQCFoL5lkkBhXhUCR5gYOQKAieYUneGZlEmJhfhqZYJFIWgQCWQp+F6GxigmHJ3BoJ4JmYMw7maKZSH6HxoAiQhvhwZ1VHYFAogmBIXiAZ55jKDIQgeChGG+G" +
"5BAoVJHiWaBYkIecznoOoeiMZYCj6IoYmcGhckeCophONoKh4aZagKLYrGkEZggeLJkCmThGDmZg5m6L4nmuOoCHaGJNnsBooAAIopCAcIZEiep2kqLwpnqCIviIBQLA" +
"4dolEECZakuK4sksYhYAgLZLOsQJLHuBBIjibR7haWYfGwawhAOJpmBuGpajuJRJkYbxAmgORynCOZvgqApejcAgIG4OYUA0e5em+PhokQXhKAYKx8AsCo+FEcJDA6D5" +
"MnwLwDkTWJulQQJuDEIphC2cQsHYbJEDCYJBAOLBrDIHwekUMBIiITgpmMcRhAOKYDgyRwnkyYwMjcK4cnAfAnCmSgzkoGhnkGZ45k6SIxjMTPIFCZOZEKSBxA0Fpui2" +
"b5LFoKIqGoaY/FKUY1gmdpQjOKxbBIV4zi0DQ6nmOhmFkUophCLILEMV5YiWCZkH+W5nl2HxfiiMwQmMTZOmqLhaLsx9iUH6JAMI1hCDjECFQawhghjBG2DseoGQzhNH" +
"wDwTAMAsgTEeCUZI1Q5jFHyLodYmWxB8GsPEVIPwxhSD6Awb4dgJD2HsAQQIoAQEB");
spSwimLane1->PutBackground(EXSWIMLANELib::exElementBackColor,RGB(255,255,255));
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Rectangular",long(0),long(0))->PutShape(EXSWIMLANELib::exShapeRectangular);
var_Elements->Add("Round",long(82),long(0))->PutShape(EXSWIMLANELib::exShapeRound);
var_Elements->Add("Rhomb",long(138),long(0))->PutShape(EXSWIMLANELib::exShapeRhomb);
var_Elements->Add("Circle",long(196),long(0))->PutShape(EXSWIMLANELib::exShapeCircle);
EXSWIMLANELib::IElementPtr var_Element = var_Elements->Add("EBN 1",long(0),long(36));
var_Element->PutBackColor(0x1000000);
var_Element->PutBorder(EXSWIMLANELib::exNoLines);
EXSWIMLANELib::IElementPtr var_Element1 = var_Elements->Add("EBN 2",long(54),long(36));
var_Element1->PutBackColor(0x2000000);
var_Element1->PutBorder(EXSWIMLANELib::exNoLines);
EXSWIMLANELib::IElementPtr var_Element2 = var_Elements->Add("EBN 3",long(108),long(36));
var_Element2->PutBackColor(0x3000000);
var_Element2->PutBorder(EXSWIMLANELib::exNoLines);
EXSWIMLANELib::IElementPtr var_Element3 = var_Elements->Add("EBN 4 ",long(160),long(36));
var_Element3->PutBackColor(0x4000000);
var_Element3->PutBorder(EXSWIMLANELib::exNoLines);
EXSWIMLANELib::IElementPtr var_Element4 = var_Elements->Add("EBN 5 ",long(220),long(36));
var_Element4->PutBackColor(0x5000000);
var_Element4->PutMinHeight(64);
var_Element4->PutMinWidth(64);
var_Element4->PutBorder(EXSWIMLANELib::exNoLines);
spSwimLane1->PutScrollPos(VARIANT_FALSE,-160);
spSwimLane1->EndUpdate();
|
21
|
Is it possible to add an inner control on the surface
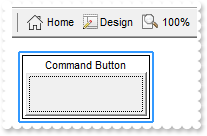
// OleEvent event - Occurs once an inside control fires an event.
void OnOleEventSwimLane1(LPDISPATCH Element,LPDISPATCH Ev)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
OutputDebugStringW( L"Ev" );
}
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
EXSWIMLANELib::IElementPtr var_Element = var_Elements->Add("activex hosting",vtMissing,vtMissing);
var_Element->PutType(EXSWIMLANELib::exElementHostControl);
var_Element->PutControl(L"Forms.CommandButton.1");
var_Element->PutCaption(L"Command Button");
var_Element->PutHeight(64);
var_Element->PutWidth(128);
var_Element->PutElementFormat(L"14;\"caption\"/\"client\"");
var_Element->PutCaptionAlign(EXSWIMLANELib::exTopCenter);
|
20
|
How can I create a copy of the element
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutBackground(EXSWIMLANELib::exElementBackColor,RGB(255,255,255));
spSwimLane1->GetElements()->Add("Element 1",long(0),long(0))->Copy(vtMissing,vtMissing)->PutCaption(L"Aka");
|
19
|
How can I define an opaque background for elements

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutBackground(EXSWIMLANELib::exElementBackColor,RGB(255,255,255));
spSwimLane1->GetElements()->Add("Element 1",long(0),long(0));
spSwimLane1->GetElements()->Add("Element 2",long(16),long(16));
|
18
|
How can I show a different context-menu for all elements
// ActionContextMenu event - Occurs when the user selects an item from the object's context menu.
void OnActionContextMenuSwimLane1(long Action,long ObjectType,VARIANT ObjectID,long CommandID,BOOL CommandChecked,VARIANT CommandCaption,VARIANT CommandValue,BOOL FAR* Cancel)
{
OutputDebugStringW( L"Action" );
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
OutputDebugStringW( L"ObjectID" );
OutputDebugStringW( L"CommandID" );
}
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutContextMenu(EXSWIMLANELib::exContextMenuElement,L"Item 1[id=1000],Item 2[id=2000]");
spSwimLane1->PutBackground(EXSWIMLANELib::exElementBackColor,RGB(255,255,255));
spSwimLane1->GetElements()->Add("Element 1",long(0),long(0));
spSwimLane1->GetElements()->Add("Element 2",long(16),long(16));
|
17
|
How can I show a different context-menu for element
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
OutputDebugStringW( _bstr_t(spSwimLane1->GetElements()->Add("Element",long(0),long(0))->InvokeContextMenu("Item 1[id=1000],Item 2[id=2000]")) );
|
16
|
How can I change the design-modes being shown on the control's toolbar
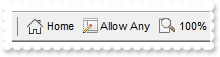
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutDesignModes(L"Read-Only#0,Allow Any#3,Only-Element#1,Only-Pool#2");
|
15
|
How can I change the default header's background color
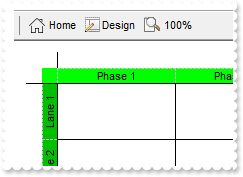
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-250),long(-150),long(500),long(250));
var_Pool->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(4);
var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren()->PutCount(4);
spSwimLane1->PutBackground(EXSWIMLANELib::exPoolHorizontalHeaderBackColor,RGB(0,255,0));
spSwimLane1->PutBackground(EXSWIMLANELib::exPoolVerticalHeaderBackColor,RGB(0,192,0));
spSwimLane1->EndUpdate();
|
14
|
How can I remove the Design item from the control's toolbar, to be locked ( no-design )
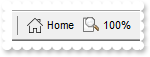
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutToolBarFormat(L"-1,100,101");
spSwimLane1->PutDesignMode(EXSWIMLANELib::exDesignLock);
|
13
|
How can I remove/disable the Color and Display-Grid from the surface's context menu
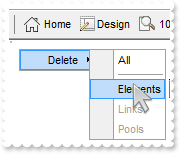
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutContextMenu(EXSWIMLANELib::exContextMenuSurface,_bstr_t("Delete[id=-32004](All[id=-32000][ttp=Deletes all the objects from the surface],[sep],Elements[id=-32001][ttp=Deletes all elemen") +
"ts from the surface],Links[id=-32002][ttp=Deletes all links from the surface],Pools[id=-32003][ttp=Deletes all pools from the su" +
"rface])");
|
12
|
How can I change the element's context-menu when the user right-clicks the element
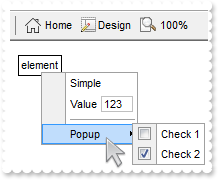
// ActionContextMenu event - Occurs when the user selects an item from the object's context menu.
void OnActionContextMenuSwimLane1(long Action,long ObjectType,VARIANT ObjectID,long CommandID,BOOL CommandChecked,VARIANT CommandCaption,VARIANT CommandValue,BOOL FAR* Cancel)
{
// ContextMenuObjectFromID(ObjectType,ObjectID).BackColor = RGB(255,255,0)
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
OutputDebugStringW( L"Action" );
OutputDebugStringW( L"CommandCaption" );
}
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
spSwimLane1->GetElements()->Add("element",long(0),long(0));
spSwimLane1->PutContextMenu(EXSWIMLANELib::exContextMenuElement,L"Simple,Value[edit=123],[sep],Popup(Check 1[chk],Check 2[chk=1])");
spSwimLane1->EndUpdate();
|
11
|
How do I prevent showing the context-menu when user right-clicks the surface
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutContextMenu(EXSWIMLANELib::exContextMenuSurface,L"");
|
10
|
How do I create parent-child (tree) lanes
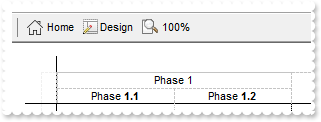
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-250),long(-150),long(500),long(250));
var_Pool->PutHeaderSize(EXSWIMLANELib::exHeaderTop,32);
var_Pool->PutHeaderVisible(EXSWIMLANELib::exHeaderBottom,VARIANT_FALSE);
EXSWIMLANELib::ILanesPtr var_Lanes = var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren();
var_Lanes->PutCount(2);
EXSWIMLANELib::ILanesPtr var_Lanes1 = var_Lanes->GetItem(long(0))->GetChildren();
var_Lanes1->PutCount(2);
var_Lanes1->GetItem(long(0))->GetHeader()->PutCaption(L"Phase <b>1.1");
var_Lanes1->GetItem(long(1))->GetHeader()->PutCaption(L"Phase <b>1.2");
spSwimLane1->EndUpdate();
|
9
|
How do I create a pool with horizontal and lanes
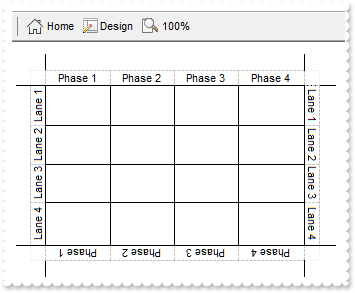
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-250),long(-150),long(500),long(250));
var_Pool->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(4);
var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren()->PutCount(4);
spSwimLane1->EndUpdate();
|
8
|
How do I add programatically a pool with horizontal lanes
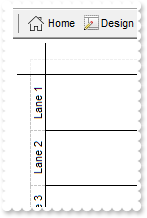
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-250),long(-150),long(500),long(250));
var_Pool->GetLane(EXSWIMLANELib::exLaneHorizontal)->GetChildren()->PutCount(4);
spSwimLane1->EndUpdate();
|
7
|
How do I add programatically a pool with vertical lanes
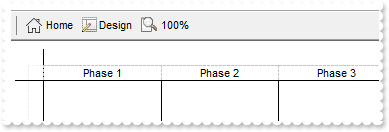
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IPoolPtr var_Pool = spSwimLane1->GetPools()->Add(long(-250),long(-150),long(500),long(250));
var_Pool->GetLane(EXSWIMLANELib::exLaneVertical)->GetChildren()->PutCount(4);
spSwimLane1->EndUpdate();
|
6
|
How can I change the toolbar's visual appearance
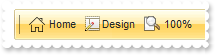
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarAppearance,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarBackColor,RGB(255,255,255));
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarForeColor,RGB(40,40,40));
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonDownBackColor,0x1606060);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonDownForeColor,RGB(240,240,240));
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonHotBackColor,0x1a0a0a0);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonHotForeColor,RGB(255,255,255));
|
5
|
How can I change the toolbar's background color
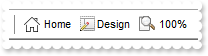
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarBackColor,RGB(255,255,255));
|
4
|
How can I fit or ensure that all elements are in the control's client area
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
EXSWIMLANELib::IElementsPtr var_Elements = spSwimLane1->GetElements();
var_Elements->Add("Element A",long(-500),long(-500))->PutBackColor(RGB(0,255,0));
var_Elements->Add("Element B",long(500),long(500))->PutBackColor(RGB(255,0,0));
var_Elements->Add("Element C",long(48),long(24));
spSwimLane1->FitToClient();
|
3
|
Context Menu - Microsoft Windows 8.1 - Ribbon Like
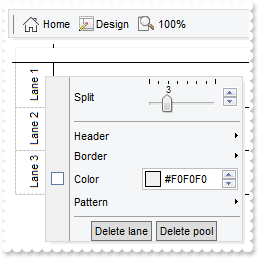
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IAppearancePtr var_Appearance = spSwimLane1->GetVisualAppearance();
var_Appearance->Add(1,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_background.ebn");
var_Appearance->Add(2,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_groupt.ebn");
var_Appearance->Add(12,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_hot.ebn");
var_Appearance->Add(14,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_select.ebn");
var_Appearance->Add(17,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_tab.ebn");
var_Appearance->Add(18,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_tabhot.ebn");
var_Appearance->Add(30,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_radio0.ebn");
var_Appearance->Add(31,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_radio1.ebn");
var_Appearance->Add(32,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_check0.ebn");
var_Appearance->Add(33,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_check1.ebn");
var_Appearance->Add(34,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_buttonu.ebn");
var_Appearance->Add(35,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSFlat-Ribbon/msfr_buttond.ebn");
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuAppearance,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolTipAppearance,0x1fefefe);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuSelBackColor,0xe000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarAppearance,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarBackColor,RGB(255,255,255));
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonHotBackColor,0xc000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonDownBackColor,0xe000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuHotBackColor,0xc000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuSelHotBackColor,0x23000000);
spSwimLane1->PutBackground(EXSWIMLANELib::BackgroundPartEnum(0x2),0x22000000);
spSwimLane1->PutBackground(EXSWIMLANELib::BackgroundPartEnum(0x3),0x23000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exCheckBoxState0,0x20000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exCheckBoxState1,0x21000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exRadioButtonState0,0x1e000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exRadioButtonState1,0x1f000000);
spSwimLane1->EndUpdate();
|
2
|
Context Menu - Microsoft Paint - Ribbon Like
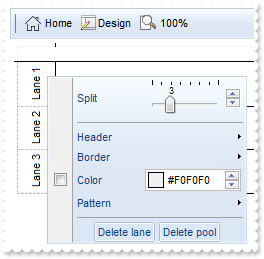
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IAppearancePtr var_Appearance = spSwimLane1->GetVisualAppearance();
var_Appearance->Add(1,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_background.ebn");
var_Appearance->Add(2,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_groupt.ebn");
var_Appearance->Add(3,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_groupo.ebn");
var_Appearance->Add(4,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_grouphot.ebn");
var_Appearance->Add(5,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_frameh.ebn");
var_Appearance->Add(6,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_framehs.ebn");
var_Appearance->Add(7,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_framehi.ebn");
var_Appearance->Add(8,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_framehe.ebn");
var_Appearance->Add(9,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_framevs.ebn");
var_Appearance->Add(10,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_framevi.ebn");
var_Appearance->Add(11,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_frameve.ebn");
var_Appearance->Add(12,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_select.ebn");
var_Appearance->Add(13,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_bordert.ebn");
var_Appearance->Add(14,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_buttond.ebn");
var_Appearance->Add(15,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_buttonu.ebn");
var_Appearance->Add(16,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_tab.ebn");
var_Appearance->Add(17,"CP:16 0 0 0 1");
var_Appearance->Add(18,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_tabhot.ebn");
var_Appearance->Add(19,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSPaint-Ribbon/mspr_tabselhot.ebn");
var_Appearance->Add(20,"CP:19 0 0 0 1");
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuAppearance,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuForeColor,RGB(21,66,139));
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuSelBackColor,0xe000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolTipAppearance,0x1fefefe);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarAppearance,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarBackColor,RGB(255,255,255));
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonHotBackColor,0xd000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonDownBackColor,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::BackgroundPartEnum(0x2),0xd000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameSingle,0x5000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameHStart,0x6000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameHIntermediate,0x7000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameHEnd,0x8000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameVStart,0x9000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameVIntermediate,0xa000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameVEnd,0xb000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuHotBackColor,0xc000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuSelHotBackColor,0xf000000);
spSwimLane1->EndUpdate();
|
1
|
Context Menu - Microsoft Office - Ribbon Like
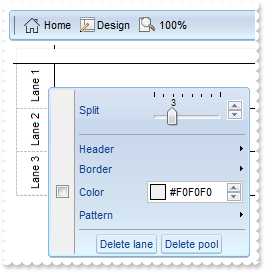
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXSWIMLANELib' for the library: 'ExSwimLane 1.0 Control Library'
#import <ExSwimLane.dll>
using namespace EXSWIMLANELib;
*/
EXSWIMLANELib::ISwimLanePtr spSwimLane1 = GetDlgItem(IDC_SWIMLANE1)->GetControlUnknown();
spSwimLane1->BeginUpdate();
EXSWIMLANELib::IAppearancePtr var_Appearance = spSwimLane1->GetVisualAppearance();
var_Appearance->Add(1,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_background.ebn");
var_Appearance->Add(2,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_groupt.ebn");
var_Appearance->Add(3,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_groupo.ebn");
var_Appearance->Add(4,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_grouphot.ebn");
var_Appearance->Add(5,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_frameh.ebn");
var_Appearance->Add(6,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_framehs.ebn");
var_Appearance->Add(7,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_framehi.ebn");
var_Appearance->Add(8,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_framehe.ebn");
var_Appearance->Add(9,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_framevs.ebn");
var_Appearance->Add(10,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_framevi.ebn");
var_Appearance->Add(11,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_frameve.ebn");
var_Appearance->Add(12,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_select.ebn");
var_Appearance->Add(13,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_bordert.ebn");
var_Appearance->Add(14,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_buttond.ebn");
var_Appearance->Add(15,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_buttonu.ebn");
var_Appearance->Add(16,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_tab.ebn");
var_Appearance->Add(17,"CP:16 0 0 0 1");
var_Appearance->Add(18,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_tabhot.ebn");
var_Appearance->Add(19,"C:/Program Files/Exontrol/ExSwimLane/Sample/EBN/MSOffice-Ribbon/msor_tabselhot.ebn");
var_Appearance->Add(20,"CP:19 0 0 0 1");
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuAppearance,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuForeColor,RGB(21,66,139));
spSwimLane1->PutBackground(EXSWIMLANELib::exToolTipAppearance,0x1fefefe);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarAppearance,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarBackColor,RGB(255,255,255));
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonHotBackColor,0xd000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exToolBarButtonDownBackColor,0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::BackgroundPartEnum(0x2),0xd000000);
spSwimLane1->PutBackground(EXSWIMLANELib::BackgroundPartEnum(0x3),0x1000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameSingle,0x5000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameHStart,0x6000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameHIntermediate,0x7000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameHEnd,0x8000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameVStart,0x9000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameVIntermediate,0xa000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuGroupPopupFrameVEnd,0xb000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuHotBackColor,0xc000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuSelBackColor,0xe000000);
spSwimLane1->PutBackground(EXSWIMLANELib::exContextMenuSelHotBackColor,0xf000000);
spSwimLane1->EndUpdate();
|